小技巧之格式化字符串输出的方式
小技巧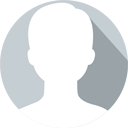
admin 发布于:2023-04-16 22:26:22
阅读:loading
格式化字符串的操作基本会非常频繁的被应用于日常开发工作中,在Java怎么做网站(“https://howtodoinjava.com/”)看到这个知识的文章后,我也花了一些时间将我曾经使用过的格式化字符串输出的实现整理了一下,这些实现似乎覆盖到了我全部的知识广度了,也特分享出来,看看我所谓的我的知识广度能有多少含金量,详见代码:
package cn.chendd.tips.examples.howtodoinjava.format;
import cn.hutool.core.util.StrUtil;
import com.sun.javafx.binding.StringFormatter;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.JUnit4;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.util.PropertyPlaceholderHelper;
import java.text.MessageFormat;
import java.util.Formatter;
import java.util.Properties;
/**
* 字符串格式化
*
* @author chendd
* @date 2023/4/16 21:03
*/
@RunWith(JUnit4.class)
public class StringFormatTest {
@Test
public void string() {
System.out.println(String.format("hello: %s,昨天是星期 %d", "chendd" , 6));
}
@Test
public void string$() {
//每个 %占位符 对应一个参数
System.out.println(String.format("hello: %s,昨天是星期 %d,是的 %s 就是我", "chendd" , 6 , "chendd"));
//每个%$ 对应一个参数索引
System.out.println(String.format("hello: %1$s,昨天是星期 %2$d,是的 %1$s 就是我", "chendd" , 6));
}
@Deprecated
@Test
public void printf$() {
//这种方法没有返回值,适用于打印输出时,而PrintStream需要 IO 流,个人不推荐
System.out.printf("hello: %1$s,昨天是星期 %2$d,是的 %1$s 就是我%n", "chendd" , 6);
}
@Test
public void formatter() {
Formatter format1 = new Formatter().format("hello: %1$s,昨天是星期 %2$d,是的 %1$s 就是我", "chendd", 6);
System.out.println(format1.out());
Formatter format2 = new Formatter().format("hello: %s,昨天是星期 %d,是的 %s 就是我", "chendd", 6 , "chendd");
System.out.println(format2.out());
}
@Test
public void stringFormatter() {
String format1 = StringFormatter.format("hello: %1$s,昨天是星期 %2$d,是的 %1$s 就是我", "chendd", 6).getValue();
System.out.println(format1);
String format2 = StringFormatter.format("hello: %s,昨天是星期 %d,是的 %s 就是我", "chendd", 6, "chendd").getValue();
System.out.println(format2);
}
@Test
public void logback() {
Logger logger = LoggerFactory.getLogger(StringFormatTest.class);
logger.info("hello: {},昨天是星期 {},是的 {} 就是我", "chendd" , 6 , "chendd");
}
@Test
public void hutool() {
String format = StrUtil.format("hello: {},昨天是星期 {},是的 {} 就是我", "chendd", 6, "chendd");
System.out.println(format);
}
@Test
public void messageFormat() {
String format = MessageFormat.format("hello: {0},昨天是星期 {1},是的 {0} 就是我", "chendd", 6);
System.out.println(format);
}
@Test
public void springFormat() {
Properties props = new Properties();
props.put("name" , "chendd");
props.put("date" , "6");
String text1 = "hello: {name},昨天是星期 {date}";
String format1 = new PropertyPlaceholderHelper("{", "}").replacePlaceholders(text1, props);
System.out.println(format1);
String text2 = "hello: ${name},昨天是星期 ${date}";
String format2 = new PropertyPlaceholderHelper("${", "}").replacePlaceholders(text2, props);
System.out.println(format2);
}
}
代码说明
(1)上述代码覆盖到了String.format函数、PrintStream、Formatter、StringFormatter、logback、hutool、messageFormat、springFormat;
(2)%s表示字符串类型的占位符,%d表示数字类的占位符,另有日期、布尔等多种占位符类型;
(3)%s表示一个参数的占位,不同位置的%s将分别占用对应出现次数的方法参数;
(4)%1$s表示获取第一个参数的占位符,该位置的参数必须为字符串类型,同一个参数可以多次被引用;
(5)Formatter和StringFormatter都是JDK内置的格式化实现,但StringFormatter为com.sun的保留包中,可能在后续的JDK版本中会被删除,建议使用前者Formatter;
(6)logback是日志API提供的额参数占位实现;
(7)hutool是国产的略有名气的工具包,提供了多个格式化的实现,如本文的StrUtil;
(8)MessageFormat是格式化文本的实现,不区分参数类型,可使用方法参数索引来占位输出;
(9)PropertyPlaceholderHelper是Spring提供的参数占位实现,可自定义占位符的前缀和后缀;
几种方式都比较简单,至于什么场景下去使用格式化多少位等可自行衡量,源码项目工程可见:“https://gitee.com/88911006/chendd-examples/tree/master/tips”。
点赞