Delphi小知识点整理
Delphi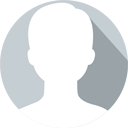
admin 发布于:2017-11-17 14:37:25
阅读:loading
'字符串以单引号囊括,逻辑等号判断用=,不等用<> ,逻辑并用and,逻辑或用or,赋值用:=
//单行注释
{}区域注释
inttostr(xxx);
strtoint(xxx);
booltoint(xxx);
等等...
showmessage('xxx');
Application.MessageBox(PChar('内容' ) , '标题' , MB_OK);
Sleep(1000);
SetCursorPos(100 , 100);//设置鼠标坐标
---------------------------------
R: TRect;
GetWindowRect(元素 , R);//获取某个元素的坐标
FormatDateTime('yyyymmddhhnn' , now);
mouse_event(MOUSEEVENTF_LEFTDOWN,0,0,0,0);
mouse_event(MOUSEEVENTF_LEFTUP,0,0,0,0);
mouse_event(MOUSEEVENTF_RIGHTDOWN,0,0,0,0);
mouse_event(MOUSEEVENTF_RIGHTUP,0,0,0,0);
WinExec(Pchar('路径) , sw_normal);
ShellExecute(Application.Handle , 'open' , Pchar('路径') , nil , nil , SW_HIDE);
UpperCase('Java');//字母转换为大写
LowerCase('Java');//字符转换为小写
copy('java' , 2 , 2);//字符串截取:从第2个位置开始,截取2个长度
length('Java');//字符串长度
trim(' Java ');//去除空格
RightStr('Java' , 2);//向右截取字符串
Pos('abc' , '1abc1');//字符串出现的位置
与Java等其他语言一样,用下标的方式进行获取,如array[0],array[1]...等
array.count为数组的长度个数
var index:Integer;
if 1 =1 then
showmessage('1=1');
//------------------------------最简单的if
if 1=1 then
begin
showmessage('ok');
end;
//------------------------------看着比较爽的if
if 1=2 then begin
showmessage('1=2');
end
else if 1=3 then begin
showmessage('1=3');
end;
//------------------------------if-else if
if 1=2 then begin
showmessage('1=2');
end
else if 1=3 then begin
showmessage('1=3');
end
else
begin
showmessage('over');
end;
//------------------------------if-else if-else
while 1=1 do begin
//TOOD
end;
for vIndex :=1 to 100 do
begin
//TODO
end;
function 函数名称(参数名:参数类型):返回值类型
//多个参数;分割,参考如下:
function FindProcess(exeFileName: String): String;
begin
Result := 'true';
end;
与function基本一致,定义改成procedure,无需返回值,区别与数据库的存储过程与自定义函数的区别
使用Result赋值返回,返回字符串如FindProcess函数;
返回数组SetLength(result , 3);
result[0] := 'A';
result[1] := 'B';
result[2] := 'C';
根据标题获取一个窗口句柄
FindWindow(nil , '窗口名称');
根据一个句柄获取子级元素句柄
FindWindowEx(上级句柄 , 0 , '类型名称' , nil);或FindWindowEx(上级句柄 , 0 , '类型名称' , '文本值');
获取同级句柄的第一个
getWindow(某句柄 , GW_HWNDFIRST);
获取同级句柄的上一个
getWindow(某句柄 , GW_HWNDPREV);
获取同级句柄的下一个
getWindow(某句柄 , GW_HWNDNEXT);
获取同级句柄的最后一个
getWindow(某句柄 , GW_HWNDLAST);
给某句柄发送鼠标按下事件
SendMessage(某句柄 , WM_LBUTTONDOWN , 0 , 0);
SendMessage(某句柄 , WM_LBUTTONUP , 0 , 0);
获取某句柄的文本值
SendMessage(某句柄 , WM_GETTEXT , 256 , integer(@p));
SendMessage(某句柄 , WM_SETTEXT , 256 , integer(pchar(文本值));
给句柄发送按键(回车键、数字键等)可触发类似密码长度校验的规则
PostMessage(某句柄 , WM_KEYDOWN , VK_RETURN , 0);
判断进程是否存在
function FindProcess(exeFileName: String): String;
var
hSnapshot: THandle;
lppe: TProcessEntry32;
Found: Boolean;
begin
Result := 'false';
hSnapshot := CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS , 0);
lppe.dwSize := SizeOf(TProcessEntry32);
Found := Process32First(hSnapshot , lppe);
while Found do
begin
if((UpperCase(ExtractFileName(lppe.szExeFile)) = UpperCase(exeFileName)) or (UpperCase(lppe.szExeFile) = UpperCase(exeFileName))) then
begin
Result := 'true';
break;
end;
Found := Process32Next(hSnapshot , lppe);
end;
end;
function EndProcess(exeFileName:String):String;
var
hSnapshot: THandle;
lppe: TProcessEntry32;
Found: Boolean;
val:integer;
findResult :String;
begin
findResult := FindProcess(exeFileName);
if findResult = 'false' then
begin
Result := findResult;
exit;
end;
val := 0;
hSnapshot := CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS , 0);
lppe.dwSize := SizeOf(TProcessEntry32);
Found := Process32First(hSnapshot , lppe);
while Integer(Found) <> 0 do
begin
if((UpperCase(ExtractFileName(lppe.szExeFile)) = UpperCase(exeFileName)) or (UpperCase(lppe.szExeFile) = UpperCase(exeFileName))) then
val := Integer(TerminateProcess(OpenProcess(PROCESS_TERMINATE, BOOL(0) , lppe.th32ProcessID) , 0));
Found := Process32Next(hSnapshot , lppe);
end;
if val = 0 then
begin
Result := 'false';
end
else begin
Result := 'true';
CloseHandle(hSnapshot);
end;
end;
procedure SwitchToWindow(hWnd:Thandle; fAltTab:boolean);stdcall;external 'User32.dll';
function SplitString(Source:String ; Deli: string):TStringList;stdcall;
var
EndOfCurrentString: byte;
StringList:TStringList;
begin
StringList:=TStringList.Create;
while Pos(Deli, Source)>0 do
begin
EndOfCurrentString := Pos(Deli, Source);
StringList.add(Copy(Source, 1, EndOfCurrentString - 1));
Source := Copy(Source, EndOfCurrentString + length(Deli), length(Source) - EndOfCurrentString);
end;
Result := StringList;
StringList.Add(source);
end;
点赞