Redis实践(4)SpringBoot Data Redis 主从架构集成
Redis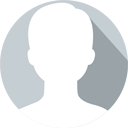
admin 发布于:2023-05-27 23:10:16
阅读:loading
前篇文章搭建了一个Redis的主从高可用架构,无论怎么玩也都是基于Redis客户端进行的,实际需要使用相关的Spring技术整合才是灵魂需要,所以基于这个一主二从的Redis环境来使用SpringBoot Data Redis来整合实践,本篇文章将搭建项目环境,配置相关参数。从Spring官网查看了SpringBoot Data Redis子项目的介绍信息,选择了2.7.12的稳定版本,比2.X再新的2.7.13-SNAPSHOT为快照版本,限制Spring Framework 5.3.27 且 JDK8,3.X的稳定版本限制的条件Spring Framework 6.0.9 且 JDK17,参考如下图所示
(2.7.12限制条件)
(3.0.6限制条件)
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<java.version>1.8</java.version>
<maven.compiler.target>1.8</maven.compiler.target>
<maven-compiler-plugin>3.8.1</maven-compiler-plugin>
<springboot.version>2.7.11</springboot.version>
</properties>
<!-- 示例导入部分坐标 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
<version>${springboot.version}</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
<version>2.11.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>${springboot.version}</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<version>${springboot.version}</version>
</dependency>
server:
port: 8080
servlet:
context-path: /
spring:
redis:
host: 192.168.244.134
port: 6379
database: 1
password: chendd
connect-timeout: 5s #连接超时时间
timeout: 5s #读取超时时间
lettuce:
pool:
enabled: true
max-active: 8 #最大连接数据库连接数,设 -1 为没有限制
max-idle: 8 #最大等待连接中的数量,设 0 为没有限制
max-wait: 10s #最大建立连接等待时间。如果超过此时间将接到异常。设为-1表示无限制。
min-idle: 0 #最小等待连接中的数量,设 0 为没有限制
time-between-eviction-runs: 10s #空闲连接回收时间
shutdown-timeout: 2s #关闭超时时间
first:
host: 192.168.244.138
port: 6379
database: 1
password: chendd
second:
host: 192.168.244.139
port: 6379
database: 1
password: chendd
【说明】
first和second为自定义的对象属性,纯粹为参数配置,需要配合自定义的参数映射并解析应用才会有意义,此处配置的spring.redis下的host、port、database、password等参数约定为master主机的配置,first和sencond将被解析为两个从机的配置。
/**
* Redis 配置类
*
* @author chendd
* @date 2023/5/16 21:30
*/
@Configuration
@ConfigurationProperties(prefix = "spring.redis")
public class RedisConfiguration {
//配置Redis Master 实例,用于Writer
@Bean(WRITE_LETTUCE_CONNECTION_FACTORY)
@Primary
public LettuceConnectionFactory writeLettuceConnectionFactory(@Autowired @Qualifier("spring.redis-org.springframework.boot.autoconfigure.data.redis.RedisProperties")
RedisProperties redisProperties) {
RedisProperties.Lettuce lettuce = redisProperties.getLettuce();
RedisProperties.Pool pool = lettuce.getPool();
GenericObjectPoolConfig poolConfig = new GenericObjectPoolConfig();
poolConfig.setMaxIdle (pool.getMaxIdle());
poolConfig.setMinIdle (pool.getMinIdle());
poolConfig.setMaxTotal(pool.getMaxActive());
poolConfig.setMaxWait(pool.getMaxWait());
LettucePoolingClientConfiguration clientConfiguration = LettucePoolingClientConfiguration.builder()
.poolConfig(poolConfig)
.commandTimeout(redisProperties.getTimeout())
.shutdownTimeout(lettuce.getShutdownTimeout())
.build();
LettuceConnectionFactory factory = new LettuceConnectionFactory(this.writeConfig(redisProperties) , clientConfiguration);
return factory;
}
//配置Redis Slave 实例,用于Read
@Bean(READ1_LETTUCE_CONNECTION_FACTORY)
public LettuceConnectionFactory read1LettuceConnectionFactory(@Autowired @Qualifier("spring.redis-org.springframework.boot.autoconfigure.data.redis.RedisProperties")
RedisProperties redisProperties) {
RedisProperties.Lettuce lettuce = redisProperties.getLettuce();
RedisProperties.Pool pool = lettuce.getPool();
GenericObjectPoolConfig poolConfig = new GenericObjectPoolConfig();
poolConfig.setMaxIdle (pool.getMaxIdle());
poolConfig.setMinIdle (pool.getMinIdle());
poolConfig.setMaxTotal(pool.getMaxActive());
poolConfig.setMaxWait(pool.getMaxWait());
LettucePoolingClientConfiguration clientConfiguration = LettucePoolingClientConfiguration.builder()
.poolConfig(poolConfig)
.commandTimeout(redisProperties.getTimeout())
.shutdownTimeout(lettuce.getShutdownTimeout())
.build();
LettuceConnectionFactory factory = new LettuceConnectionFactory(this.read1Config() , clientConfiguration);
factory.setShareNativeConnection(false);
return factory;
}
//配置Redis Slave 实例,用于Read
@Bean(READ2_LETTUCE_CONNECTION_FACTORY)
public LettuceConnectionFactory read2LettuceConnectionFactory(@Autowired @Qualifier("spring.redis-org.springframework.boot.autoconfigure.data.redis.RedisProperties")
RedisProperties redisProperties) {
RedisProperties.Lettuce lettuce = redisProperties.getLettuce();
RedisProperties.Pool pool = lettuce.getPool();
GenericObjectPoolConfig poolConfig = new GenericObjectPoolConfig();
poolConfig.setMaxIdle (pool.getMaxIdle());
poolConfig.setMinIdle (pool.getMinIdle());
poolConfig.setMaxTotal(pool.getMaxActive());
poolConfig.setMaxWait(pool.getMaxWait());
LettucePoolingClientConfiguration clientConfiguration = LettucePoolingClientConfiguration.builder()
.poolConfig(poolConfig)
.commandTimeout(redisProperties.getTimeout())
.shutdownTimeout(lettuce.getShutdownTimeout())
.build();
LettuceConnectionFactory factory = new LettuceConnectionFactory(this.read2Config() , clientConfiguration);
factory.setShareNativeConnection(false);
return factory;
}
}
【说明】
(1)上述代码为部分示例代码,并不完整,定义了三个Redis的接入实例,等同于接入了主从架构的一主二从;
(2)Master主节点为Writer,写入数据节点;Read1和Read2均为Slave,读取数据节点;
(3)writeConfig、read1Config、read2Config的示例构建均使用了RedisStandaloneConfiguration单击版本的示例配置;
点赞
发表评论
评论列表
留言区
- Redis实践(1)简单介绍与安装
- Redis实践(2)客户端介绍
- Redis实践(3)主从复制高可用架构
- Redis实践(4.1)SpringBoot 测试主从RedisTemplate
- Redis实践(4.2)SpringBoot 测试主从连接池
- Redis实践(4.3)SpringBoot 读模式的负载均衡
- Redis实践(4.4)SpringBoot 测试主从读写分离
- Redis实践(4.5)SpringBoot 测试主从数据类型读写
- Redis实践(4.6)SpringBoot 测试主从数据序列化
- Redis实践(5)Cluster模式高可用架构
- Redis实践(6)SpringBoot Data Redis Cluster架构集成
- Redis实践(7)Redisson 简单介绍
- Redis实践(8)Redisson 简单实践