itext导出PDF表格文档的简单应用
itext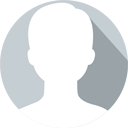
admin 发布于:2022-07-09 22:54:54
阅读:loading
本篇文章是对于itext组件(Java读写PDF文档的开源实现,非Apache托管)的简单整理,主要是将个人曾在工作中使用到的技能实现予以整理,实际上截止目前为止对于PDF文档的读取几乎没有特别实现,但是对于解析PDF的实现略有所知,基本上也是使用itext组件基于特定的文本段落或者表格行列等特定位置来做特定的读取解析,但是对于PDF文档中本身就图片的内容也仅能读取未图片,若想读取到图片的内容还需要进一步的OCR识别,不再多说。而对于PDF文档的生成记忆深刻的也就一次而已,本篇文章将整理出当时生成PDF文档(主要是表格数据报表的导出)的细节知识点整理汇总,若如今再来实现PDF文档的生成则首先肯定不是本篇文章中使用的纯itext组件,而是itext项目的子项目html2pdf,它里面提供了大量的基于html生成pdf文档的实现案例以及各种应用场景下的知识点细节;或者使用本站特别推荐的docx4j项目,先生成docx文档,再将docx文档转换为PDF文档(同样也支持各种复杂的样式效果和图片等),当然操作PDF的开源实现还有一款pdfbox的实现,但由于几年前曾曾略有了解后认为入门级门槛较高,社区活跃度稍低等原因最终没有过多去了解。
(1)单元格合并;
(2)文本居中;
(3)文本居左;
(4)边框细线;
(5)超链接;
(6)文本颜色、下划线;
(7)文档标题、作者、主题、关键字;
package cn.chendd.itext;
import ...;
/**
* 简单生成Pdf测试类
*
* @author chendd
* @date 2022/7/6 22:26
*/
public class Test {
public static void main(String[] args) {
Document doc = null;
PdfWriter writer = null;
try {
File file = File.createTempFile("用户数据_", ".pdf");
List<User> dataList = createDataList();
doc = new Document(PageSize.A4, 10, 10, 10, 10);
writer = PdfWriter.getInstance(doc, new FileOutputStream(file));
BaseFont baseFont = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", BaseFont.NOT_EMBEDDED);
Font headerFont = new Font(baseFont, 12F, Font.BOLD);//标题
Font titleFont = new Font(baseFont, 10F, Font.BOLD);//列明
Font contentFont = new Font(baseFont, 8F, Font.NORMAL);//内容
doc.open();
//主题信息
doc.addAuthor("chendd");
doc.addTitle("用户数据");
doc.addSubject("家有儿女");//数据表格
addTableContent(doc, headerFont, titleFont, contentFont, dataList);
System.out.println("文档创建完毕:" + file.getAbsolutePath());
} catch (Exception e) {
e.printStackTrace();
} finally {
if (doc != null) {
doc.close();
}
if (writer != null) {
writer.close();
}
if (doc != null) {
doc.close();
}
}
}
//添加主信息列表的相关表格数据
static void addTableContent(Document doc, Font headerFont, Font titleFont, Font contentFont, List<User> dataList) throws Exception {
PdfPTable table = new PdfPTable(7);// 6列的表格
table.setWidths(new int[]{50, 100, 100, 100, 100, 150, 150});
table.setWidthPercentage(100);//百分比的宽度
PdfPCell titleCell = new PdfPCell(new Paragraph(new Chunk("用户信息表", headerFont)
.setLocalDestination("用户信息表_link")));
titleCell.setMinimumHeight(38f);
titleCell.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
titleCell.setVerticalAlignment(PdfPCell.ALIGN_MIDDLE);
titleCell.setColspan(7);
PdfPRow titleRow = new PdfPRow(new PdfPCell[]{titleCell, titleCell, titleCell, titleCell, titleCell, titleCell, titleCell});
table.getRows().add(titleRow);
//添加字段名称行
PdfPRow headerRow = new PdfPRow(new PdfPCell[]{
new SimplePdfCell("序号", titleFont, 18F).getCenterCell(),
new SimplePdfCell("名称", titleFont, 18F).getCenterCell(),
new SimplePdfCell("性别", titleFont, 18F).getCenterCell(),
new SimplePdfCell("年龄", titleFont, 18F).getCenterCell(),
new SimplePdfCell("身份证号码", titleFont, 18F).getCenterCell(),
new SimplePdfCell("联系地址", titleFont, 18F).getCenterCell(),
new SimplePdfCell("操作", titleFont, 18F).getCenterCell()
});
table.getRows().add(headerRow);
//超链接字体样式
Font linkFont = new Font(contentFont.getBaseFont(), contentFont.getSize());
linkFont.setColor(42, 0, 255);//蓝色
linkFont.setStyle(Font.UNDERLINE);//下划线
for (int i = 0, size = dataList.size(); i < size; i++) {
User user = dataList.get(i);
PdfPRow dataRow = new PdfPRow(new PdfPCell[]{
new SimplePdfCell(user.getUserId().toString(), contentFont, 18F).getCenterCell(),
new SimplePdfCell(user.getUserName(), contentFont, 18F).getLeftCell(),
new SimplePdfCell(user.getSex(), contentFont, 18F).getCenterCell(),
new SimplePdfCell(user.getAge().toString(), contentFont, 18F).getCenterCell(),
new SimplePdfCell(user.getIdNumber(), contentFont, 18F).getCenterCell(),
new SimplePdfCell(user.getAddress(), contentFont, 18F).getLeftCell(),
new SimplePdfCell(new Paragraph(new Chunk("回到顶部", linkFont).setLocalGoto("用户信息表_link")),
contentFont, 18F).getCenterCell()
});
table.getRows().add(dataRow);
}
doc.add(table);
doc.newPage();
}
/**
* 构造100条数据
* return数据列表
*/
private static List<User> createDataList() {
List<User> dataList = new ArrayList<User>();
for (int i = 1; i <= 100; i++) {
User user = new User();
dataList.add(user);
user.setUserId(i);
boolean flag = i % 2 == 0;
user.setUserName(flag ? "chenzy" : "chenyt");
user.setSex(flag ? "女" : "男");
user.setAge((short) (flag ? 6 : 1));
user.setIdNumber(flag ? "420682" : "420115");
user.setAddress(flag ? "湖北省老河口市" : "湖北省武汉市");
}
return dataList;
}
}
源码工程只是简单的Poi示例,代码比较少同样也比较简单,下载地址为:源码下载.zip;
点赞