quartz在普通Java中的使用
quartz定时任务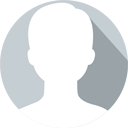
admin 发布于:2017-06-08 09:53:17
阅读:loading
现在使用定时任务太普遍了,以前使用都是集成了spring环境,使用起来简单配置一下即可,功能强大,易于维护,这次工作中需要做一款小软件程序,并未集成spring,考虑使用jdk自带的Timer或者是使用quartz组件,由于现在常用的技术平台中也是使用到了quartz组件,也是基于包装后的使用(类似于普通Java类的方式调用),而非与spring集成,于是我就准备也使用Java普通类的方式进行使用。这里所说的Java普通类的含义是想表明非集成框架的方式,如果你也需要这种基于普通类的方式调用,那么这里你值得拥有,东西比较简单,直接上代码。
我们可在http://www.quartz-scheduler.org/;下载,下载来的文件包里面有一些例子,可以打开瞅上一眼,基本都是围绕创建调度工厂再创建一个调度任务,然后执行一个任务的逻辑处理,下文中我也是围绕只创建一个调度工厂的方式进行封装,参考如下:
根据实验发现需要quartz-2.2.3.jar、quartz-jobs-2.2.3.jar、slf4j-api-1.7.7.jar、slf4j-log4j12-1.7.7.jar、jta-1.1.jar log4j-1.2.17.jar、commons-logging-1.1.1.jar,最后两个文件是log4j的日志文件。
由于本次程序最终支出打包成exe的方式,而配置类的文件需要支持手工修改,所以第一该配置文件存储的文件夹目录与src目录同级,并非常规的在src目录下;第二读取的配置文件目录同样支持中文特殊字符等路径规则;后文中的代码同样基于这种规则编写。
该文件描述了调度工厂实例、线程数、任务文件等,参考代码如下:
#===============================================================
#Configure Main Scheduler Properties
#===============================================================
#实例名
org.quartz.scheduler.instanceName = QuartzScheduler
#实例ID
org.quartz.scheduler.instanceId = AUTO
#===============================================================
#Configure ThreadPool
#===============================================================
org.quartz.threadPool.class = org.quartz.simpl.SimpleThreadPool
#线程个数
org.quartz.threadPool.threadCount = 2
org.quartz.threadPool.threadPriority = 5
#===============================================================
#Configure JobStore
#===============================================================
org.quartz.jobStore.misfireThreshold = 60000
org.quartz.jobStore.class = org.quartz.simpl.RAMJobStore
#JobInitializationPlugin
org.quartz.plugin.jobIitializer.class = org.quartz.plugins.xml.XMLSchedulingDataProcessorPlugin
org.quartz.plugin.jobIitializer.fileNames = config/quartz_jobs.xml
org.quartz.plugin.jobIitializer.failOnFileNotFound = true
#多长时间检查更新一次参数,单位:秒
org.quartz.plugin.jobIitializer.scanInterval = 3600
#org.quartz.plugin.jobIitializer.overWriteExistingJobs = true
#org.quartz.plugin.jobIitializer.wrapInUserTransation = false
#org.quartz.threadPool.threadsInheritContextClassLoaderOfInitializingThread = true
该文件声明了所有的定时任务相关
<?xml version="1.0" encoding="UTF-8"?>
<job-scheduling-data version="1.8"
xmlns="http://www.quartz-scheduler.org/xml/JobSchedulingData"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.quartz-scheduler.org/xml/JobSchedulingData ;
http://www.quartz-scheduler.org/xml/job_scheduling_data_1_8.xsd">
<schedule>
<job>
<name>JOB_HELLO</name>
<group>JOB_GROUP_HELLO</group>
<description>Hello定时任务</description>
<job-class>demo.jobs.HelloJob</job-class>
<!-- 其他自定义参数 -->
</job>
<trigger>
<cron>
<name>TASKJOB_TRIGGER_HELLO</name>
<group>TRIGGER_GROUP_HELLO</group>
<job-name>JOB_HELLO</job-name>
<job-group>JOB_GROUP_HELLO</job-group>
<cron-expression>00 0/1 * * * ?</cron-expression>
</cron>
</trigger>
</schedule>
</job-scheduling-data>
调度工厂类
package cn.chendd.quartz;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.quartz.JobListener;
import org.quartz.Scheduler;
import org.quartz.SchedulerException;
import org.quartz.SchedulerFactory;
import org.quartz.impl.StdSchedulerFactory;
import cn.chendd.util.PropertiesUtil;
/**
* <pre>
* 定时任务的简单封装
* </pre>
*/
public class QuartzManage {
private SchedulerFactory schedulerFactory;//quartz对象
private static QuartzManage quartzManage;//单例类
private Log log = LogFactory.getLog(getClass());
private QuartzManage(){
PropertiesUtil quartzProps = new PropertiesUtil("quartz.properties");
try {
schedulerFactory = new StdSchedulerFactory(quartzProps.getProperties());
} catch (SchedulerException e) {
log.error(e);
}
}
public static QuartzManage getInstance() {
if(quartzManage == null){
synchronized (QuartzManage.class) {
if(quartzManage == null){
quartzManage = new QuartzManage();
}
}
}
return quartzManage;
}
/**
* <pre>
* 启动定时任务
* </pre>
*/
public void start() {
try {
Scheduler scheduler = this.getScheduler();
boolean started = scheduler.isStarted();
if(started == false){
scheduler.start();
}
} catch (SchedulerException e) {
log.error(e);
}
}
/**
* <pre>
* 添加监听器
* </pre>
* @param jobListener
*/
public QuartzManage addJobListener(JobListener jobListener){
Scheduler scheduler = this.getScheduler();
try {
scheduler.getListenerManager().addJobListener(jobListener);
} catch (SchedulerException e) {
log.error(e);
}
return this;
}
/**
* <pre>
* 关闭定时任务
* </pre>
*/
public void shutdown(){
try {
Scheduler scheduler = this.getScheduler();
boolean shutdown = scheduler.isShutdown();
if(shutdown == false){
scheduler.shutdown(true);
}
} catch (SchedulerException e) {
log.error(e);
}
}
/**
* <pre>
* 获取定时调度
* </pre>
* @return
*/
protected Scheduler getScheduler() {
try {
return schedulerFactory.getScheduler();
} catch (SchedulerException e) {
log.error(e);
}
return null;
}
}
读取配置文件的工具类
package cn.chendd.util;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
public class PropertiesUtil {
private String fileName;
public PropertiesUtil(String fileName){
this.fileName = fileName;
}
public String getPropertiesByPath() {
String userDir = System.getProperty("user.dir") + File.separator + "config"
+ File.separator + fileName;
return userDir;
}
public boolean hasConfigProperties(){
String path = getPropertiesByPath();
File file = new File(path);
return file.exists();
}
public Properties getProperties(){
boolean exist = hasConfigProperties();
Properties props = new Properties();
if(! exist){
return props;
}
InputStream in = null;
try {
String path = getPropertiesByPath();
File file = new File(path);
in = new FileInputStream(file);
props.load(in);
} catch (Exception e) {
e.printStackTrace();
} finally {
if(in != null){
try {
in.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return props;
}
}
任务类
package demo.jobs;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.quartz.Job;
import org.quartz.JobExecutionContext;
import org.quartz.JobExecutionException;
/**
* 具体的任务调度
*/
public class HelloJob implements Job {
@Override
public void execute(JobExecutionContext arg0) throws JobExecutionException {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
System.out.println("当前时间:" + sdf.format(new Date()));
}
}
运行类
package demo.jobs;
import cn.chendd.quartz.QuartzManage;
public class Demo {
public static void main(String[] args) throws Exception {
QuartzManage quartzManage = QuartzManage.getInstance();
System.out.println("启动定时任务");
quartzManage.start();
Thread.sleep(3600L);
quartzManage.shutdown();//销毁定时任务
}
}
点赞